mirror of
https://github.com/open-goal/jak-project.git
synced 2024-10-21 07:37:45 -04:00
Fixes decal on tfrag: 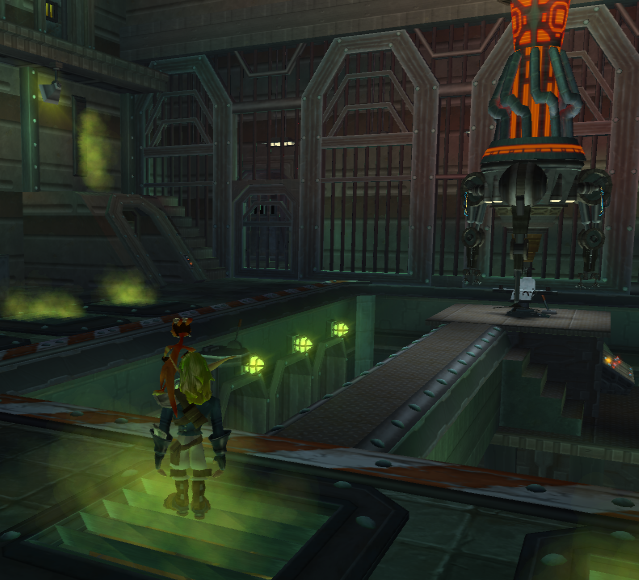 Sets up jak 2 alpha shrub test settings. They are still too dark, but there's no longer incorrect alpha test: 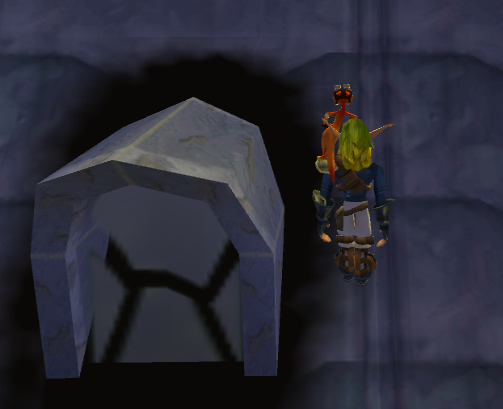 Fix decal on shrub, a feature used in exactly one place in jak 1: 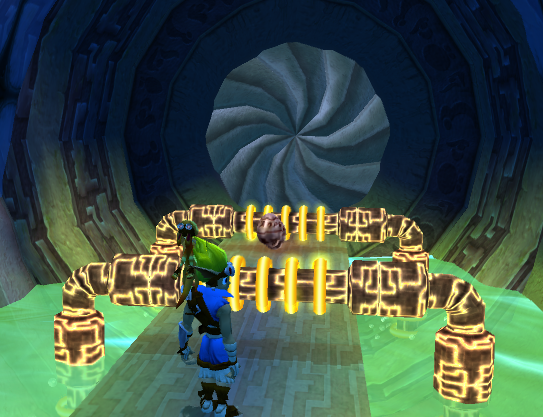 Fixed issue with u16 overflow in castle on the alpha channel, causing flickering. It barely overflowed, which made me suspicious that we had some error somewhere. But I think that there code is robust against overflows.
83 lines
2.2 KiB
GLSL
83 lines
2.2 KiB
GLSL
#version 430 core
|
|
|
|
layout (location = 0) in vec3 position_in;
|
|
layout (location = 1) in vec3 tex_coord_in;
|
|
layout (location = 2) in vec3 rgba_base;
|
|
layout (location = 3) in int time_of_day_index;
|
|
|
|
uniform vec4 hvdf_offset;
|
|
uniform mat4 camera;
|
|
uniform float fog_constant;
|
|
uniform float fog_min;
|
|
uniform float fog_max;
|
|
uniform int decal;
|
|
layout (binding = 10) uniform sampler1D tex_T1; // note, sampled in the vertex shader on purpose.
|
|
|
|
out vec4 fragment_color;
|
|
out vec3 tex_coord;
|
|
out float fogginess;
|
|
|
|
void main() {
|
|
|
|
|
|
// old system:
|
|
// - load vf12
|
|
// - itof0 vf12
|
|
// - multiply with camera matrix (add trans)
|
|
// - let Q = fogx / vf12.w
|
|
// - xyz *= Q
|
|
// - xyzw += hvdf_offset
|
|
// - clip w.
|
|
// - ftoi4 vf12
|
|
// use in gs.
|
|
// gs is 12.4 fixed point, set up with 2048.0 as the center.
|
|
|
|
// the itof0 is done in the preprocessing step. now we have floats.
|
|
|
|
// Step 3, the camera transform
|
|
vec4 transformed = -camera[3];
|
|
transformed -= camera[0] * position_in.x;
|
|
transformed -= camera[1] * position_in.y;
|
|
transformed -= camera[2] * position_in.z;
|
|
|
|
// compute Q
|
|
float Q = fog_constant / transformed.w;
|
|
|
|
// do fog!
|
|
fogginess = 255 - clamp(-transformed.w + hvdf_offset.w, fog_min, fog_max);
|
|
|
|
// perspective divide!
|
|
transformed.xyz *= Q;
|
|
// offset
|
|
transformed.xyz += hvdf_offset.xyz;
|
|
// correct xy offset
|
|
transformed.xy -= (2048.);
|
|
// correct z scale
|
|
transformed.z /= (8388608);
|
|
transformed.z -= 1;
|
|
// correct xy scale
|
|
transformed.x /= (256);
|
|
transformed.y /= -(128);
|
|
// hack
|
|
transformed.xyz *= transformed.w;
|
|
// scissoring area adjust
|
|
transformed.y *= SCISSOR_ADJUST * HEIGHT_SCALE;
|
|
gl_Position = transformed;
|
|
|
|
// time of day lookup
|
|
// start with the vertex color (only rgb, VIF filled in the 255.)
|
|
fragment_color = vec4(rgba_base, 1);
|
|
// get the time of day multiplier
|
|
vec4 tod_color = texelFetch(tex_T1, time_of_day_index, 0);
|
|
// combine
|
|
fragment_color *= tod_color * 4;
|
|
fragment_color.a *= 2;
|
|
|
|
if (decal == 1) {
|
|
fragment_color.xyz = vec3(1.0, 1.0, 1.0);
|
|
}
|
|
|
|
tex_coord = tex_coord_in;
|
|
tex_coord.xy /= 4096;
|
|
}
|